Difference between revisions of "RxJava"
Jump to navigation
Jump to search
(→Links) |
|||
(33 intermediate revisions by the same user not shown) | |||
Line 1: | Line 1: | ||
+ | {{:Self_Driving_RC_Car/Links}} | ||
+ | |||
+ | = github = | ||
* https://github.com/ReactiveX/RxJava | * https://github.com/ReactiveX/RxJava | ||
= Versions = | = Versions = | ||
Line 5: | Line 8: | ||
* https://mvnrepository.com/artifact/io.reactivex.rxjava3/rxjava | * https://mvnrepository.com/artifact/io.reactivex.rxjava3/rxjava | ||
* https://github.com/ReactiveX/RxJava/wiki/What's-different-in-3.0 | * https://github.com/ReactiveX/RxJava/wiki/What's-different-in-3.0 | ||
+ | <uml> | ||
+ | hide circle | ||
+ | package io { | ||
+ | package reactivex { | ||
+ | package rjxjava3 { | ||
+ | package core { | ||
+ | note top of Observer | ||
+ | see [[http://reactivex.io/RxJava/3.x/javadoc/io/reactivex/rxjava3/core/Observer.html javadoc]] | ||
+ | end note | ||
+ | interface Observer { | ||
+ | void onComplete() | ||
+ | void onError(@NonNull Throwable e) | ||
+ | void onNext(T t) | ||
+ | void onSubscribe(@NonNull Disposable d) | ||
+ | } | ||
+ | } | ||
+ | } | ||
+ | } | ||
+ | } | ||
+ | </uml> | ||
== RxJava 2 == | == RxJava 2 == | ||
Line 10: | Line 33: | ||
* https://mvnrepository.com/artifact/io.reactivex.rxjava2/rxjava | * https://mvnrepository.com/artifact/io.reactivex.rxjava2/rxjava | ||
* https://github.com/ReactiveX/RxJava/wiki/What%27s-different-in-2.0 | * https://github.com/ReactiveX/RxJava/wiki/What%27s-different-in-2.0 | ||
+ | * https://blog.kaush.co/2017/06/21/rxjava1-rxjava2-migration-understanding-changes/ | ||
+ | * https://howtoprogram.xyz/2017/02/07/how-to-create-observable-in-rxjava-2/ | ||
+ | <uml> | ||
+ | hide circle | ||
+ | package org { | ||
+ | package reactivestreams { | ||
+ | note top of Subscriber | ||
+ | Will receive call to onSubscribe(Subscription) | ||
+ | once after passing an instance of | ||
+ | Subscriber to Publisher.subscribe(Subscriber). | ||
+ | |||
+ | No further notifications will be received | ||
+ | until Subscription.request(long) is called. | ||
+ | see [[https://www.reactive-streams.org/reactive-streams-1.0.0-javadoc/org/reactivestreams/Subscriber.html javadoc]] | ||
+ | end note | ||
+ | interface Subscriber { | ||
+ | void onComplete() | ||
+ | void onError(java.lang.Throwable t) | ||
+ | void onNext(T t) | ||
+ | void onSubscribe(Subscription s) | ||
+ | } | ||
+ | note top of Subscription | ||
+ | A Subscription represents a | ||
+ | one-to-one lifecycle of a Subscriber | ||
+ | subscribing to a Publisher. | ||
+ | |||
+ | It can only be used once by a single Subscriber. | ||
+ | |||
+ | It is used to both signal desire for data | ||
+ | and cancel demand (and allow resource cleanup). | ||
+ | see [[https://www.reactive-streams.org/reactive-streams-1.0.0-javadoc/org/reactivestreams/Subscription.html javadoc]] | ||
+ | end note | ||
+ | interface Subscription { | ||
+ | void cancel() | ||
+ | void request(long n) | ||
+ | } | ||
+ | } | ||
+ | } | ||
+ | package io { | ||
+ | package reactivex { | ||
+ | note top of Observable | ||
+ | The Observable class is the non-backpressured, | ||
+ | optionally multi-valued base reactive class that | ||
+ | offers factory methods, intermediate operators and | ||
+ | the ability to consume synchronous | ||
+ | and/or asynchronous reactive dataflows. | ||
+ | see [[http://reactivex.io/RxJava/2.x/javadoc/io/reactivex/Observable.html javadoc]]. | ||
+ | end note | ||
+ | class Observable { | ||
+ | Disposable subscribe(Consumer<? super T> onNext) | ||
+ | } | ||
+ | note top of Observer | ||
+ | Provides a mechanism for receiving | ||
+ | push-based notifications see [[http://reactivex.io/RxJava/2.x/javadoc/io/reactivex/Observer.html javadoc]]. | ||
+ | end note | ||
+ | interface Observer { | ||
+ | void onComplete() | ||
+ | void onError(Throwable e) | ||
+ | void onNext(T t) | ||
+ | void onSubscribe(Disposable d) | ||
+ | } | ||
+ | package disposables { | ||
+ | note top of Disposable | ||
+ | Represents a disposable resource | ||
+ | see[[http://reactivex.io/RxJava/2.x/javadoc/io/reactivex/disposables/Disposable.html javadoc]]. | ||
+ | end note | ||
+ | interface Disposable { | ||
+ | void dispose() | ||
+ | boolean isDisposed() | ||
+ | } | ||
+ | } | ||
+ | } | ||
+ | } | ||
+ | </uml> | ||
== RxJava 1 == | == RxJava 1 == | ||
1.0.0 was released 2014-11 | 1.0.0 was released 2014-11 | ||
* https://mvnrepository.com/artifact/io.reactivex/rxjava | * https://mvnrepository.com/artifact/io.reactivex/rxjava | ||
+ | <uml> | ||
+ | hide circle | ||
+ | package rx { | ||
+ | note top of Observable | ||
+ | The Observable class that | ||
+ | implements the Reactive Pattern. | ||
+ | see [[http://reactivex.io/RxJava/javadoc/rx/Observable.html javadoc]] | ||
+ | end note | ||
+ | interface Observable { | ||
+ | Subscription subscribe() | ||
+ | } | ||
+ | note top of Observer | ||
+ | Provides a mechanism | ||
+ | for receiving push-based notifications see [[http://reactivex.io/RxJava/javadoc/rx/Observer.html javadoc]] | ||
+ | end note | ||
+ | interface Observer { | ||
+ | void onCompleted() | ||
+ | void onError(java.lang.Throwable e) | ||
+ | void onNext(T t) | ||
+ | } | ||
+ | note top of Subscriber | ||
+ | Provides a mechanism | ||
+ | for receiving push-based notifications | ||
+ | from Observables, and permits manual | ||
+ | unsubscribing from these Observables | ||
+ | see [[http://reactivex.io/RxJava/javadoc/rx/Subscriber.html javadoc]]. | ||
+ | end note | ||
+ | interface Subscriber { | ||
+ | void add(Subscription s) | ||
+ | boolean isUnsubscribed() | ||
+ | void onStart() | ||
+ | protected void request(long n) | ||
+ | void setProducer(Producer p) | ||
+ | void unsubscribe() | ||
+ | } | ||
+ | note top of Subscription | ||
+ | Subscription returns from | ||
+ | Observable.subscribe(Subscriber) | ||
+ | to allow unsubscribing | ||
+ | see [[http://reactivex.io/RxJava/javadoc/rx/Observer.html javadoc]]. | ||
+ | end note | ||
+ | interface Subscription { | ||
+ | boolean isUnsubscribed() | ||
+ | void unsubscribe() | ||
+ | } | ||
+ | } | ||
+ | </uml> | ||
+ | |||
+ | = Links = | ||
+ | * https://vertx.io/docs/vertx-rx/java/ | ||
+ | * https://github.com/ReactiveX/RxJava/wiki/How-To-Use-RxJava | ||
+ | * http://reactivex.io/RxJava/javadoc/io/reactivex/Observable.html | ||
+ | * https://github.com/ReactiveX/RxJava/wiki/Backpressure | ||
+ | * [https://medium.com/@jagsaund/5-not-so-obvious-things-about-rxjava-c388bd19efbc Not so obvious things about rxjava] | ||
+ | * https://github.com/ReactiveX/RxJava/wiki/Creating-Observables | ||
+ | * http://akarnokd.blogspot.com/2016/03/operator-fusion-part-1.html | ||
+ | * https://www.littlerobots.nl/blog/Note-to-self-RxJava-SyncOnSubscribe/ | ||
+ | * https://pedroo21.github.io/RxJava/ | ||
+ | * https://github.com/Froussios/Intro-To-RxJava/blob/master/Part%203%20-%20Taming%20the%20sequence/6.%20Hot%20and%20Cold%20observables.md | ||
+ | * https://proandroiddev.com/understanding-rxjava-subscribeon-and-observeon-744b0c6a41ea | ||
+ | |||
+ | = WhatLinksHere = | ||
+ | {{WhatLinksHere}} |
Latest revision as of 17:29, 2 March 2020
Click here to comment see Self Driving RC Car
github
Versions
RxJava 3
3.0.0 was released 2020-02-14
- https://mvnrepository.com/artifact/io.reactivex.rxjava3/rxjava
- https://github.com/ReactiveX/RxJava/wiki/What's-different-in-3.0
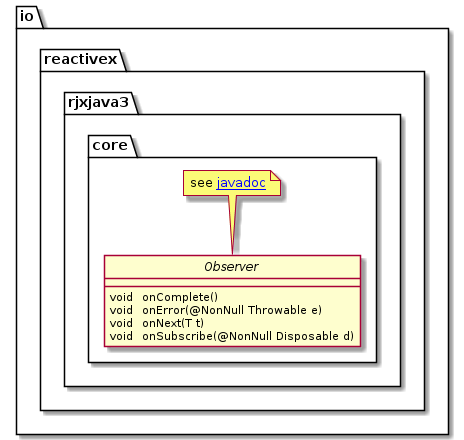
RxJava 2
2.0.0 was released 2016-11-29 there will be bugfixes until 2021-02-28
- https://mvnrepository.com/artifact/io.reactivex.rxjava2/rxjava
- https://github.com/ReactiveX/RxJava/wiki/What%27s-different-in-2.0
- https://blog.kaush.co/2017/06/21/rxjava1-rxjava2-migration-understanding-changes/
- https://howtoprogram.xyz/2017/02/07/how-to-create-observable-in-rxjava-2/
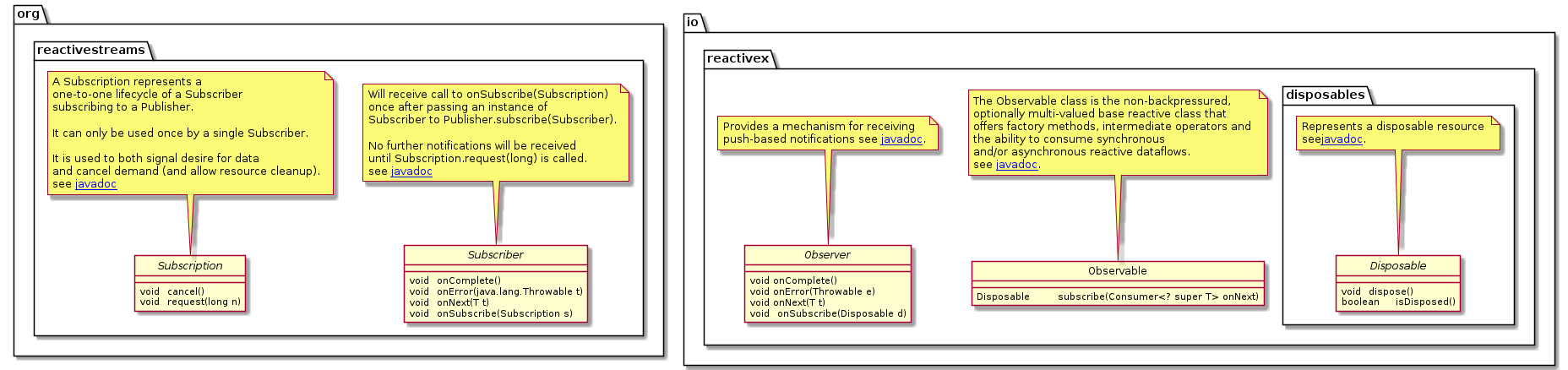
RxJava 1
1.0.0 was released 2014-11
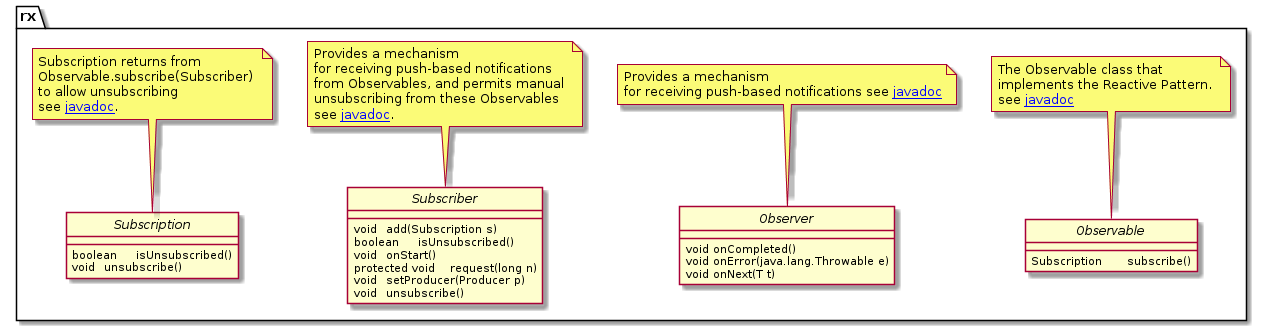
Links
- https://vertx.io/docs/vertx-rx/java/
- https://github.com/ReactiveX/RxJava/wiki/How-To-Use-RxJava
- http://reactivex.io/RxJava/javadoc/io/reactivex/Observable.html
- https://github.com/ReactiveX/RxJava/wiki/Backpressure
- Not so obvious things about rxjava
- https://github.com/ReactiveX/RxJava/wiki/Creating-Observables
- http://akarnokd.blogspot.com/2016/03/operator-fusion-part-1.html
- https://www.littlerobots.nl/blog/Note-to-self-RxJava-SyncOnSubscribe/
- https://pedroo21.github.io/RxJava/
- https://github.com/Froussios/Intro-To-RxJava/blob/master/Part%203%20-%20Taming%20the%20sequence/6.%20Hot%20and%20Cold%20observables.md
- https://proandroiddev.com/understanding-rxjava-subscribeon-and-observeon-744b0c6a41ea